- How to Use Laravel Sanctum to Authenticate Your SPA or Mobile App
- Understanding Laravel Sanctum: A Brief Overview
- API Token Authentication
- How It Works
- Use Cases
- What features are provided by a sanctum?
- Advantages of Sanctum
- Optimizing Your SPA or Mobile App with Laravel Sanctum
- Conclusion
How to Use Laravel Sanctum to Authenticate Your SPA or Mobile App
Single Page Applications (SPAs), mobile applications, and simple token-based APIs are all targeted by the lightweight authentication framework offered by Laravel Sanctum. Within Sanctum’s scope, every application user gains the capability to generate multiple API tokens, each affiliated with their account. These tokens can be endowed with particular abilities or scopes, dictating the specific actions they are authorized to execute. Sanctum offers a straightforward solution for generating API tokens for your users, eliminating the complexities of OAuth. This functionality draws inspiration from platforms like GitHub and other applications that distribute “personal access tokens”.
For example, imagine that your application’s “account settings” have a screen where a user can generate an API token for their account. Sanctum can be employed to create and oversee these tokens. Tokens normally have a long expiration; however, they can be manually revoked at any time. Laravel Sanctum provides the capability to centrally store user API tokens within a dedicated database table. It enables the authentication of incoming HTTP requests by validating the authorization header, which should contain a valid API token. The handling of API authentication within your Laravel application will be secure and seamless thanks to this strategy.
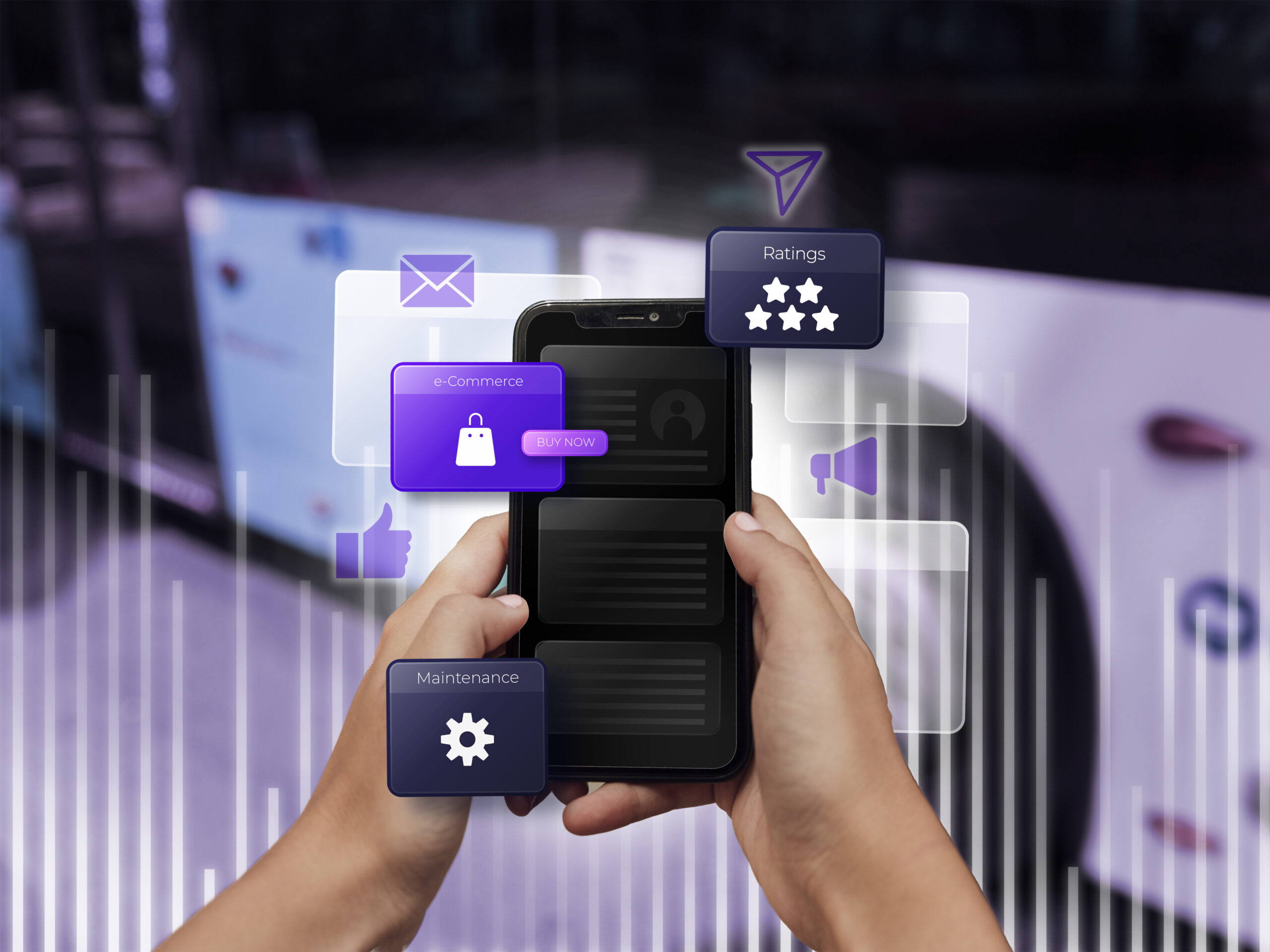
Understanding Laravel Sanctum: A Brief Overview
Before we dive into the details of implementation, let’s grasp the essence of Laravel Sanctum. It’s a package specifically designed for Laravel applications that enable developers to effortlessly set up token-based authentication systems. Whether you’re building a React-based SPA or a native mobile app, Sanctum offers a flexible and secure way to manage user authentication.
User Registration and Login
Begin by enabling user registration and login functionalities in your SPA or mobile app. Utilise Sanctum’s token-based authentication to securely manage user sessions. Through a series of API endpoints, your application can register new users, log them in, and issue access tokens for subsequent requests.
Token Generation and Management
When a user successfully logs in, your application should generate an access token using Sanctum. This token serves as a digital signature, granting the user permission to access protected routes and resources. Implement token management logic to refresh tokens, log users out, and revoke tokens when necessary.
Creating Protected Routes
Secure specific routes and endpoints within your SPA or mobile app using Sanctum’s middleware. By applying the auth:sanctum middleware to these routes, you ensure that only authenticated users with valid tokens can access the associated resources. This adds an extra layer of security to sensitive parts of your application.
Stateless Authentication
Laravel Sanctum operates in a stateless manner, which means that each request must include the access token for verification. This approach eliminates the need for server-side sessions, making your application more scalable and efficient. Ensure that your frontend application stores and sends the token with every request to maintain continuous authentication.
Revoking Tokens
In situations where you need to revoke a user’s access or log them out, Laravel Sanctum provides mechanisms to invalidate tokens. By revoking a token, you prevent its further use, effectively logging the user out. This feature is particularly useful in scenarios where a user’s device is lost or compromised.
Cross-Origin Requests
For SPAs and mobile apps, dealing with cross-origin requests is crucial. Laravel Sanctum simplifies this process by automatically handling CORS (Cross-Origin Resource Sharing) headers. This ensures that your front end can communicate with the backend API without facing CORS-related obstacles.
Customization and Configuration
Laravel Sanctum offers a range of customization options to tailor the authentication process according to your application’s needs. From token expiration durations to customizing response messages, you have the flexibility to shape the authentication flow to align with your project’s requirements.
API Token Authentication
Issuing API Tokens
With Sanctum, you can generate API tokens or personal access tokens that serve as authentication credentials for API requests to your application. When making API requests with these tokens, it is important to include the token as a bearer token in the authorization header of the request.
Token Abilities
Sanctum allows you to assign “abilities” to tokens. Capabilities serve a similar purpose to OAuth’s “scopes”.
Protecting Routes
To guarantee the authentication and safeguarding of all incoming requests, you can incorporate the sanctum authentication guard into the routes found within both the routes/web.php and routes/api.php route files. This will require authentication to access those routes.
Revoking Tokens
You can revoke tokens by deleting them from your database. You can accomplish this by leveraging the token relationship furnished by the Laravel\Sanctum\HasApiTokens property. Through this relationship, you can eliminate tokens from the database, effectively revoking their access.
Token Expiration
By default, Sanctum tokens have no expiration date and can only be invalidated by revoking them. In case you have configured an expiration time for tokens in your application, consider scheduling a task to automatically eliminate tokens that have expired.
How It Works
Laravel Sanctum exists to solve two separate problems. Before delving further into the intricacies of the library, let’s explore each of these aspects in detail.
API Tokens
Sanctum is a simple package you may use to issue API tokens to your users without the complications of OAuth. This feature draws inspiration from platforms like GitHub and other applications that issue “personal access tokens.” For example, imagine the “account settings” of your application have a screen where A user can generate an API token associated with their account. You may use Sanctum to generate and manage those tokens. These tokens generally possess an extensive expiration period, often spanning years. However, users retain the authority to manually revoke these tokens whenever they deem necessary.
Laravel Sanctum provides this functionality through the storage of user API tokens in a unified database table. The authentication of incoming HTTP requests is accomplished via the authorization header, which is expected to include a valid API token.
SPA Authentication
Sanctum serves its purpose by presenting a straightforward method for authenticating single-page applications (SPAs) that necessitate interaction with a Laravel-fueled API. These SPAs may live in the same repository as your Laravel application or they may reside in a completely separate repository, such as an SPA created using the Vue CLI or a Next.js application.
Regarding this functionality, Sanctum abstains from utilizing any form of tokens. Instead, Sanctum uses Laravel’s built-in cookie-based session authentication services. In most cases, Sanctum utilizes Laravel’s web authentication guard to achieve this purpose. This approach offers the advantages of CSRF (Cross-Site Request Forgery) protection and session-based authentication, along with guarding against the inadvertent exposure of authentication credentials through cross-site scripting (XSS) vulnerabilities.
Sanctum exclusively endeavors to authenticate via cookies solely when the incoming request stems from the front end of your Single Page Application (SPA). When scrutinizing an incoming HTTP request, Sanctum’s initial step involves searching for an authentication cookie. In cases where no such cookie exists, Sanctum subsequently inspects the authorization header for the presence of an authentication token.
Use Cases
Creating RESTful APIs in PHP with Laravel
Empowers each user within your application to create multiple API tokens associated with their account.
The Sanctum package provides developers with the capability to establish multi-authentication systems for their websites.
It assists developers in crafting authentication systems that are both secure and efficient.
Authenticate any HTTP request.
What features are provided by a sanctum?
Laravel Sanctum provides the following features:
The Sanctum authentication guard guarantees the authentication of incoming requests.
Sanctum authentication presents the benefits of CSRF protection, session-based authentication, and a shield against potential XSS attacks that might otherwise expose authentication data.
You can also use Sanctum tokens to authenticate requests from your mobile application to your API.
It safeguards routes by requiring authentication for all incoming requests.
Advantages of Sanctum
Laravel Sanctum provides the following advantages:
Sanctum is a user-friendly package that grants you the ability to provide API tokens to your users, all while sidestepping the intricate intricacies often linked with OAuth implementations.
Sanctum offers the flexibility of both session-based and token-based authentication, making it well-suited for authenticating single-page applications (SPAs).
Sanctum enables the sharing of session cookies between your API and SPA, as long as they are hosted on the same root domain.
Sanctum is fully capable of meeting your requirements, eliminating the need for the additional burden associated with the more intricate setup of a Passport.
Optimizing Your SPA or Mobile App with Laravel Sanctum
Enhancing Security with Laravel Sanctum
When it comes to safeguarding your single-page application (SPA) or mobile app, using Laravel Sanctum is a strategic choice. This section will dive into how this package enhances the security of your application.
Secure Token Management
With Laravel Sanctum, token management becomes a breeze. Tokens act as keys, granting users access to the safeguarded assets of your application. These tokens are stored securely and verified by the server, guaranteeing that solely authenticated users are granted entry. This layer of security effectively thwarts unauthorized access and safeguards valuable user data from compromise.
Seamless Multi-Device Access
In today’s world, users access applications across various devices. Laravel Sanctum facilitates this by enabling seamless multi-device access. After a user logs in and acquires an access token, they can effortlessly transition between devices without the necessity for repetitive logins. This user-centric attribute elevates the user experience while upholding a strong security foundation.
Role-Based Access Control
Not all users require the same level of access within an application. Laravel Sanctum allows you to implement role-based access control (RBAC). Through the allocation of roles to users and the subsequent limitation of their access by these roles, you possess the ability to meticulously customize the capabilities of every user within your application. This is particularly useful for applications with varying user privileges.
Leveraging Laravel Sanctum’s Flexibility
Token Expiry and Refresh/
Tokens provided by Laravel Sanctum have expiration periods. This introduces an additional layer of security, ensuring that in the event of a token compromise, its validity remains confined to a restricted time frame. However, renewing tokens can be seamless for users. By implementing token refresh mechanisms, users can stay logged in without frequent logins, while the application maintains security through token expiration.
Stateful Authentication for Specific Routes
While Laravel Sanctum promotes stateless authentication, there might be cases where you need stateful authentication, particularly for certain routes. With the Sanctum::actingAs() method, you can authenticate as a specific user for specific routes, allowing for a balance between stateful and stateless authentication as per your application’s requirements.
Token Revocation and Logouts
Tokens can be revoked using Laravel Sanctum, which is especially important in scenarios where a user logs out or their device is lost. Revoking tokens promptly ensures that unauthorized access is prevented. Moreover, Laravel Sanctum offers a simple and efficient way to log users out, enhancing the security of your app’s user sessions.
Implementing Laravel Sanctum: Best Practises
Regular Updates and Maintenance
Just like any other component in your application, keeping Laravel Sanctum up-to-date is crucial. Regularly updating the package ensures that you have the latest security patches and enhancements. This practice minimizes vulnerabilities and keeps your authentication system robust.
Error Handling and Reporting
Implement comprehensive error handling and reporting when working with Laravel Sanctum. Clear error messages and appropriate HTTP status codes provide meaningful feedback to users and developers alike. This not only enhances the user experience but also aids in troubleshooting potential issues.
Monitoring User Activity
Leverage Laravel Sanctum to monitor user activity and track usage patterns. This can help identify unusual behavior that might indicate security breaches or unauthorized access. By keeping a close eye on user activity, you can respond swiftly to potential threats and maintain the integrity of your application.
Conclusion
In conclusion, implementing secure authentication mechanisms for your SPA or mobile app is a critical aspect of software development. Laravel Sanctum emerges as a dependable solution, seamlessly integrating into your Laravel project and providing an efficient way to handle token-based authentication. You can confidently utilize Laravel Sanctum to improve the security of your applications while guaranteeing a positive user experience by following the instructions provided in this article. The need for user data protection and authentication must not change as the digital landscape changes, and Laravel Sanctum is a platform that gives developers the tools they need to do precisely that.